How To Run Thread Program In Dev C++
- To compile and run simple console applications such as those used as examples in these tutorials it is enough with opening the file with Dev-C and hit F11. As an example, try: File - New - Source File (or Ctrl.
- Sep 06, 2016 In this article I'm going to present a gentle and modernized introduction to multi-threaded and parallel programming. While there are no concrete examples in this overview, I'm going to cover the general concepts and terminology, as well as an overview of the tools available to you as a developer to leverage multi-threaded techniques in our.
May 26, 2010 Learn to create your first C program using Dev-C.
You don't have to compile it from the command prompt. In fact in the dev environment click the compile button (not the compile AND run button) and that will give you an executable. Then all you need to do navigate to that path using cd c:dev and run your program from there. With MSVC, there are several ways to program with multiple threads: You can use C/WinRT and the Windows Runtime library, the Microsoft Foundation Class (MFC) library, C/CLI and the.NET runtime, or the C run-time library and the Win32 API. This article is about multithreading in C. For example code, see Sample multithread program in C. Remote Development. VS Code and the C extension support Remote Development allowing you to work over SSH on a remote machine or VM, inside a Docker container, or in the Windows Subsystem for Linux (WSL). To install support for Remote Development: Install the VS Code Remote Development Extension Pack. A multithreaded program contains two or more elements that can run concurrently. Each part of such a program is called the thread, and each thread defines a separate path of execution. Multithreading support was introduced in C+11. The std::thread is the thread class that describes a single thread in C.
- C++ Basics
- C++ Object Oriented
- C++ Advanced
- C++ Useful Resources
- Selected Reading
Multithreading is a specialized form of multitasking and a multitasking is the feature that allows your computer to run two or more programs concurrently. In general, there are two types of multitasking: process-based and thread-based.
Process-based multitasking handles the concurrent execution of programs. Thread-based multitasking deals with the concurrent execution of pieces of the same program.
A multithreaded program contains two or more parts that can run concurrently. Each part of such a program is called a thread, and each thread defines a separate path of execution.
C++ does not contain any built-in support for multithreaded applications. Instead, it relies entirely upon the operating system to provide this feature.
This tutorial assumes that you are working on Linux OS and we are going to write multi-threaded C++ program using POSIX. POSIX Threads, or Pthreads provides API which are available on many Unix-like POSIX systems such as FreeBSD, NetBSD, GNU/Linux, Mac OS X and Solaris.
Creating Threads
The following routine is used to create a POSIX thread − /little-snitch-release-263.html.
Here, pthread_create creates a new thread and makes it executable. This routine can be called any number of times from anywhere within your code. Here is the description of the parameters −
Sr.No | Parameter & Description |
---|---|
1 | thread An opaque, unique identifier for the new thread returned by the subroutine. |
2 | attr An opaque attribute object that may be used to set thread attributes. You can specify a thread attributes object, or NULL for the default values. |
3 | start_routine The C++ routine that the thread will execute once it is created. |
4 | arg A single argument that may be passed to start_routine. It must be passed by reference as a pointer cast of type void. NULL may be used if no argument is to be passed. |
The maximum number of threads that may be created by a process is implementation dependent. Once created, threads are peers, and may create other threads. There is no implied hierarchy or dependency between threads.
Terminating Threads
There is following routine which we use to terminate a POSIX thread −
Here pthread_exit is used to explicitly exit a thread. Typically, the pthread_exit() routine is called after a thread has completed its work and is no longer required to exist.
If main() finishes before the threads it has created, and exits with pthread_exit(), the other threads will continue to execute. Otherwise, they will be automatically terminated when main() finishes.
Example
This simple example code creates 5 threads with the pthread_create() routine. Each thread prints a 'Hello World!' message, and then terminates with a call to pthread_exit().
Compile the following program using -lpthread library as follows −
Now, execute your program which gives the following output −
Passing Arguments to Threads
This example shows how to pass multiple arguments via a structure. You can pass any data type in a thread callback because it points to void as explained in the following example −
When the above code is compiled and executed, it produces the following result −
Joining and Detaching Threads
There are following two routines which we can use to join or detach threads −
The pthread_join() subroutine blocks the calling thread until the specified 'threadid' thread terminates. When a thread is created, one of its attributes defines whether it is joinable or detached. Only threads that are created as joinable can be joined. If a thread is created as detached, it can never be joined.
This example demonstrates how to wait for thread completions by using the Pthread join routine.
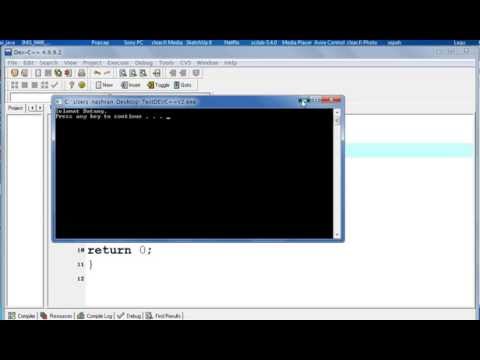
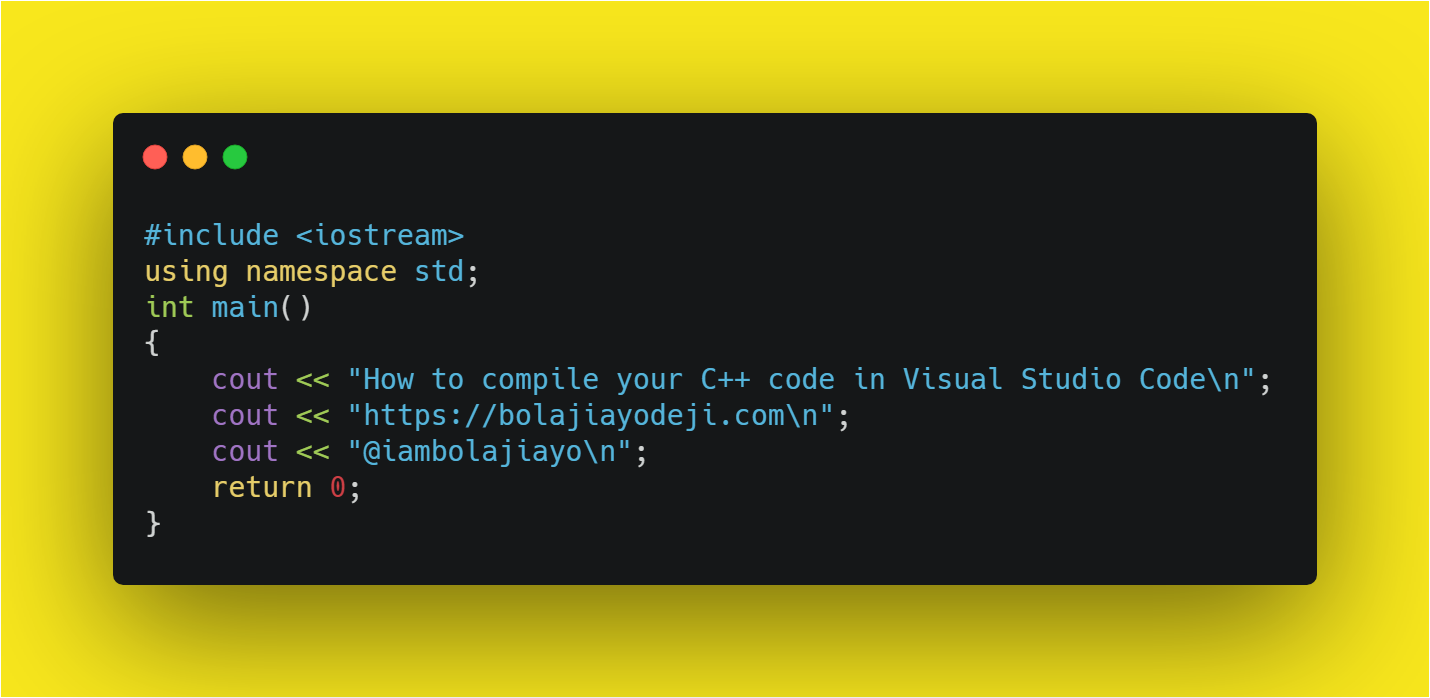
When the above code is compiled and executed, it produces the following result −
Well i use Dev-c++ 4.9.9.2 for programming.i m using it from last two years..recently i learned java and saw that all java programs are run through command prompt. I tried tha same in c++. i saved my program in dev-cpp/bin .but i am not able to do this.what is the exact procedure please tell me. and How to pass command line argument. Is there any limitation on the type of arguments passed to main. like i saw somewhere int main(int argc,string args[])
- 7 Contributors
- forum 9 Replies
- 2,658 Views
- 4 Years Discussion Span
- commentLatest Postby joaquinceLatest Post
vmanes1,165
Here's a couple links to command line argument help:
http://malun1.mala.bc.ca:8080/~wesselsd/csci161/notes/args.html
'>http://www.cprogramming.com/tutorial/lesson14.html
As to running your program, be sure you opening the command window in the folder where the .exe file is located. That's the file you execute as in
If you are unfamiliar with working at the command prompt, here's some help:
http://www.claymania.com/dos-primer.html
Dev C++ Program Examples
Val